19th April 2019 • 10 min read
ReasonML for production React Apps? (Part 1)
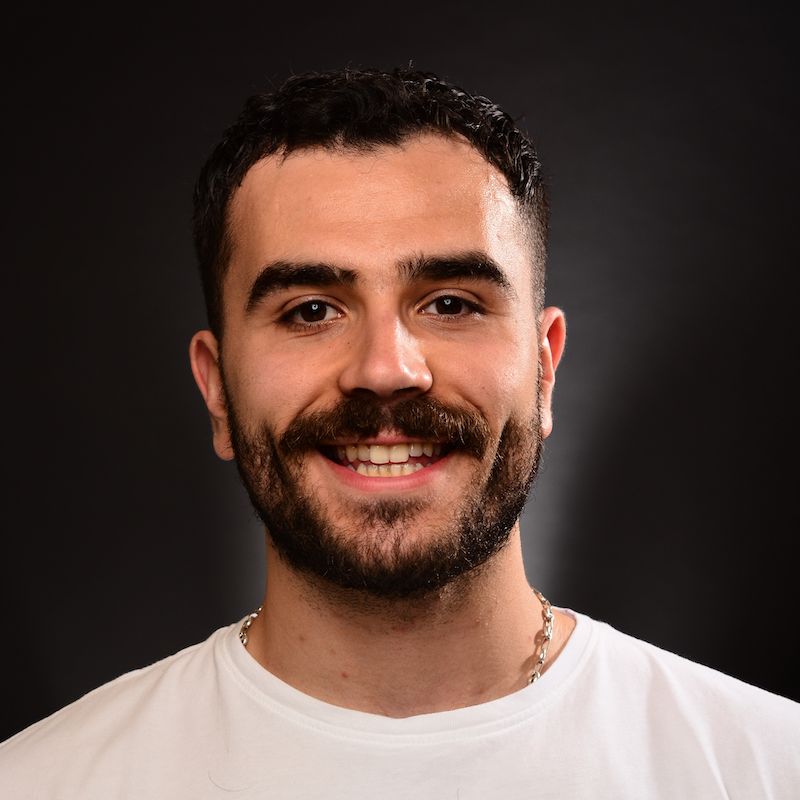
Seif Ghezala
ReasonML is a functional programming language with smartly inferred strict types, that compiles to JavaScript. ReasonReact is Reason bindings for ReactJS (aka the translated ReasonML version of the ReactJS). It has improved a lot lately and even added support for hooks in a release a couple of days ago.
In this series of articles, I will build applications in ReasonReact and try to accomplish most of the tasks I usually do with ReactJS. For each article, I will share what I like/dislike about building React applications in Reason. The goal is to determine how ready is ReasonML for building serious React applications.
Access Part 2 here, Part 3 here and Part 4 here.
I decided to start with a simple application. We will build a small words counter with the following features:
There is an input where I can write text.
There is a word count that updates while I write text.
There is a button to clear text.
There is a button to copy text.
You can find the final source code here. Since we will build the application in iterations, there is a branch for each iteration.
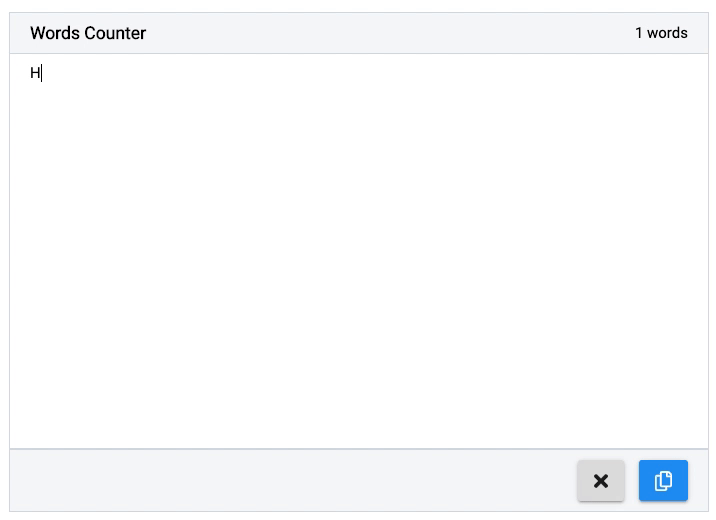
Final result
First, let's download the Reason to JavaScript compiler bs-platform (BuckleScript):
1npm install -g bs-platform
The package comes with bsb, a CLI tool to quickly bootstrap a Reason project based on a template. Let's generate our project based on the react-hooks template:
1bsb -init words-counter -theme react-hooks
Let's also use VSCode as our code editor, and download reason-vscode. This is the editor plugin officially recommended by ReasonML.
To take advantage of the formatting feature, let's enable the Format on Save option in the editor's settings:
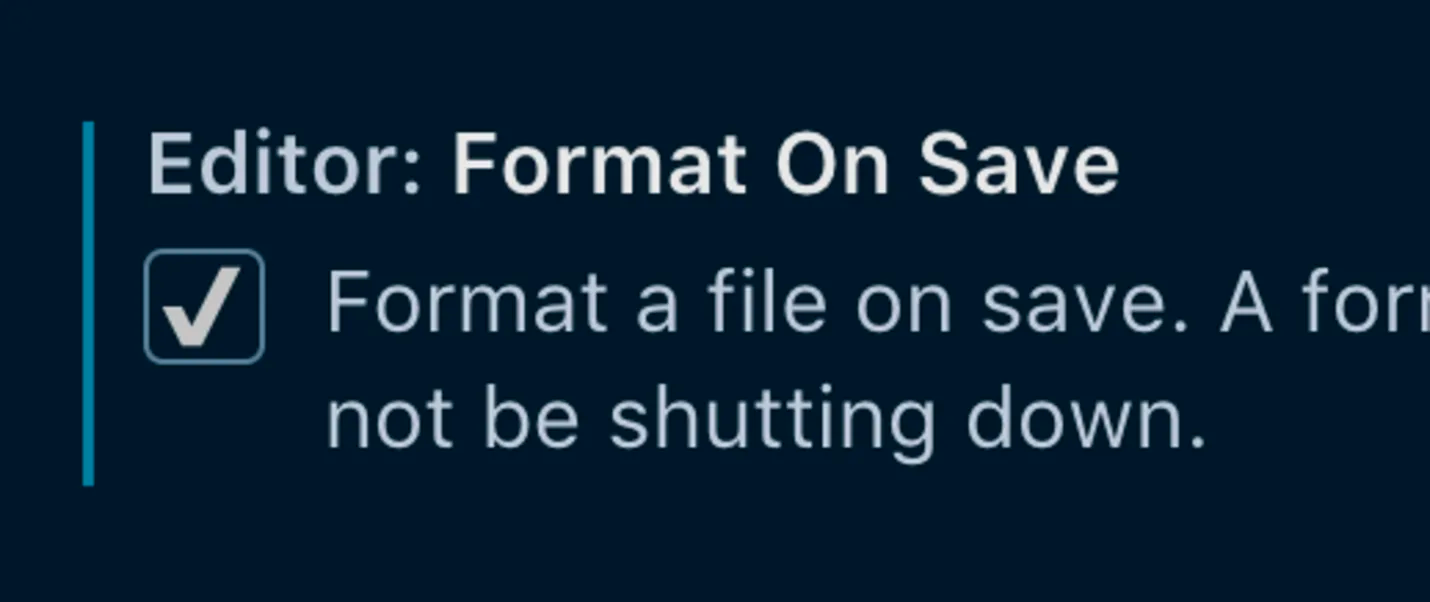
Enabling the Format On Save option on VSCode
I like
The getting-started experience is very good. The BuckleScript build tool (bsb) is a much faster version of create-react-app or yeoman.
The Editor tooling is also great:
It formats the code style and syntax (just like configuring ESLint with Prettier).
It also provides information about types when hovering on values.
In this first iteration, we just want to have a nice text area with a title to write text and store it in a state variable:

Iteration #1: there is an input where I can write text
1/* src/App.re */
2
3[%bs.raw {|require('./App.css')|}];
4
5[@react.component]
6let make = () => {
7 let (text, setText) = React.useState(() => "");
8
9 let handleTextChange = e => ReactEvent.Form.target(e)##value |> setText;
10
11 <div className="App">
12 <div className="header">
13 <h3> {"Words Counter" |> ReasonReact.string} </h3>
14 </div>
15 <textarea
16 placeholder="Express yourself..."
17 value=text
18 onChange=handleTextChange
19 />
20 </div>;
21};
I dislike
Accessing the target value of a form event is a bit of overhead.
Having to use
ReasonReact.string
with everystring
value needs some getting used to, even if the composition operator|>
helps a bit.useState
requires a function. Although this is useful when making an expensive initial state computation, it's unnecessary in most cases. I would have preferred having the 2 forms of this hook (one that accepts a value, and one that accepts a function) with different names.
I like
It was pretty easy to get started with a simple app with CSS. Although the syntax for requiring a CSS file is a bit weird, the whole experience is still great.
DOM elements are fully typed, which has 2 benefits:
You can know before runtime whether you assigned a wrong value to a prop: no more typos! It's like having propTypes built-in for the attributes of all the DOM elements.
DOM elements are self-documenting. You can instantly hover on an element to see the possible attributes it accepts (no need to Google them anymore).
Iteration #2: there is a word count that updates while I write text
In this iteration, we want to show a count of the words typed so far:

Iteration #2: there is a word count that updates while I write text
First, let's create a function that returns the number of words in a string input:
1let countWordsInString = text => {
2 let spacesRegex = Js.Re.fromString("\s+");
3
4 switch (text) {
5 | "" => 0
6 | noneEmptyText =>
7 noneEmptyText
8 |> Js.String.trim
9 |> Js.String.splitByRe(spacesRegex)
10 |> Js.Array.length
11 };
12};
So here's what the function does:
If the text is empty, we just return 0.
Otherwise, we just trim the text and use
Js.String.splitByRe
to split it by the regular expression\s+
(which basically means 1 or more spaces followed by any character) and return the length of the array we obtain.
1/* src/App.re */
2
3[%bs.raw {|require('./App.css')|}];
4
5let countWordsInString = text => {
6 let spacesRegex = Js.Re.fromString("\s+");
7
8 switch (text) {
9 | "" => 0
10 | noneEmptyText =>
11 noneEmptyText
12 |> Js.String.trim
13 |> Js.String.splitByRe(spacesRegex)
14 |> Js.Array.length
15 };
16};
17
18[@react.component]
19let make = () => {
20 let (text, setText) = React.useState(() => "");
21
22 let handleTextChange = e => ReactEvent.Form.target(e)##value |> setText;
23
24 let wordsCountText =
25 (text |> countWordsInString |> string_of_int) ++ " words";
26
27 <div className="App">
28 <div className="header">
29 <h3> {"Words Counter" |> ReasonReact.string} </h3>
30 <span> {ReasonReact.string(wordsCountText)} </span>
31 </div>
32 <textarea
33 placeholder="Express yourself..."
34 value=text
35 onChange=handleTextChange
36 />
37 </div>;
38};
I like
Reason's smart inference is great:
Although I didn't provide any type annotations, the
countWordsInString
function is self-documenting. Hovering over it shows that it accepts astring
and returns anint
.At some point, I returned the split array from
countWordsInString
instead of its length. I was able to catch that bug at build time before even looking at the application in the browser.
Iteration #3: there is a button to clear text
In this iteration, we want to have a button to clear text:

Iteration #3: there is a button to clear textLet's add a button to clear the text in the textarea
Iteration #3: there is a button to clear textLet's add a button to clear the text in the textarea
In JavaScript, I use the svgr Webpack loader to import SVG icons as React components directly from their corresponding .svg
files.
Since imports are typed in Reason, I decided to have an icon in the clear button to see how painful it would be to import SVG icons as React components.
Since we will have another button in the next iteration which will look differently (spoiler alert), let's have our button as a separate component and make it have two categories for styling purposes:
PRIMARY: blue button
SECONDARY: gray button
1/* src/Button.re */
2
3[%bs.raw {|require('./Button.css')|}];
4
5type categoryT =
6 | SECONDARY
7 | PRIMARY;
8
9let classNameOfCategory = category =>
10 "Button "
11 ++ (
12 switch (category) {
13 | SECONDARY => "secondary"
14 | PRIMARY => "primary"
15 }
16 );
17
18[@react.component]
19let make =
20 (
21 ~onClick,
22 ~title: string,
23 ~children: ReasonReact.reactElement,
24 ~disabled=false,
25 ~category=SECONDARY,
26 ) => {
27 <button onClick className={category |> classNameOfCategory} title disabled>
28 children
29 </button>;
30};
To use svgr, let's add the following rule in the Webpack module
configuration:
1{
2 test: /\.svg$/,
3 use: ['@svgr/webpack'],
4}
In JavaScript, we can import an svg component by doing this:
1import { ReactComponent as Times } from "./times";
2
Since Webpack applies svgr to the JavaScript resulting from compiling our Reason source code, we just need to make BuckleScript translate our Reason import into a named es6 import.
To do so, we first have to configure /bs-config.json
(the configuration file for the BuckleScript compiler) to use es6 imports:
1 "package-specs": [
2 {
3 "module": "es6",
4 "in-source": true
5 }
6 ],
ReasonReact make
function compiles to a JavaScript React component! This means that if we want to use a component "Foo" that is written in JavaScript, all that we have to do is: 1- Create the component in Reason. 2- Import the JS component as the make
function of the Reason component and annotate its props.
So in the module Foo.re
, we would have the following:
1[@bs.module "./path/to/Foo.js"][@react.component]
2external make: (~someProp: string, ~someOtherProp: int) => React.element = "default";
Which means ... that we can use that to import an SVG component with svgr! Let's use it to import the ./times.svg
icon and just annotate the height
prop since it's the only one we will be using:
1[@bs.module "./times.svg"] [@react.component]
2external make: (~height: string) => React.element = "default";
Our ReasonReact components were automatically considered as modules because we created them in separate files (Button.re, App.re). Since the Times component is pretty small (2 lines), we can use Reason's module syntax to create it:
1/* src/App.re */
2
3[%bs.raw {|require('./App.css')|}];
4
5let countWordsInString = text => {
6 let spacesRegex = Js.Re.fromString("\s+");
7
8 switch (text) {
9 | "" => 0
10 | noneEmptyText =>
11 noneEmptyText
12 |> Js.String.trim
13 |> Js.String.splitByRe(spacesRegex)
14 |> Js.Array.length
15 };
16};
17
18module Times = {
19 [@bs.module "./times.svg"] [@react.component]
20 external make: (~height: string) => React.element = "default";
21};
22
23[@react.component]
24let make = () => {
25 let (text, setText) = React.useState(() => "");
26
27 let handleTextChange = e => ReactEvent.Form.target(e)##value |> setText;
28
29 let handleClearClick = _ => setText(_ => "");
30
31 let wordsCountText =
32 (text |> countWordsInString |> string_of_int) ++ " words";
33
34 <div className="App">
35 <div className="header">
36 <h3> {"Words Counter" |> ReasonReact.string} </h3>
37 <span> {ReasonReact.string(wordsCountText)} </span>
38 </div>
39 <textarea
40 placeholder="Express yourself..."
41 value=text
42 onChange=handleTextChange
43 />
44 <div className="footer">
45 <Button
46 title="Clear text"
47 onClick=handleClearClick
48 disabled={String.length(text) === 0}>
49 <Times height="20px" />
50 </Button>
51 </div>
52 </div>;
53};
I dislike
If I want to make a reusable button that should accept all the attributes a native DOM button does, I would have to list all of those attributes. In JavaScript, I can avoid that by just using the spread operation:
1function Button(props) {
2 return <button {...props} />;
3}
However, ReasonReact doesn't allow the spread operator. (I wonder if there is a way to achieve what I want with ReasonReact 🤔)
I like
The ability to specify the type of children is very powerful. This is possible with PropTypes in JavaScript but very limited compared to Reason. We can, for example, specify that the component only accepts 2 children (as a tuple).
Variants were useful to categorize buttons. Categorizing components is something that occurs very often, so being able to do that with an actual reliable type instead of string constants is a huge win.
Using the Webpack svgr plugin to import an SVG as a component was actually pretty painless. It's very simple and yet ensures type safety since we have to annotate the types.
Iteration #4: there is a button to copy text
In this iteration, we want to have a button to copy text to the clipboard:
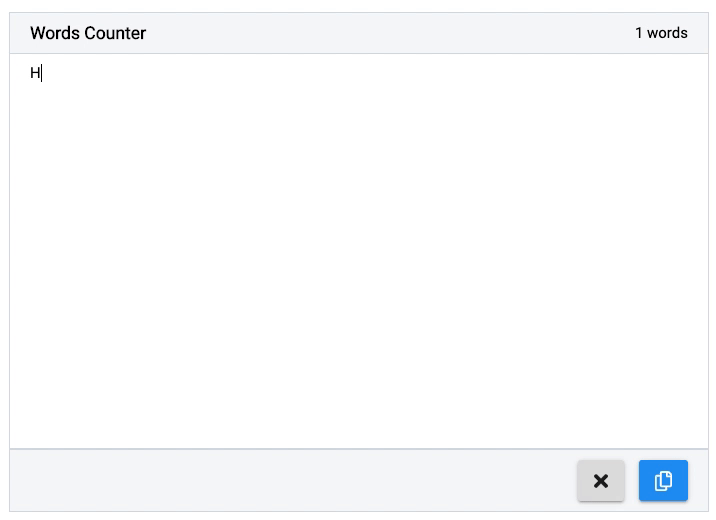
Iteration #4: there is a button to copy text
To do so, I want to use react-copy-to-clipboard, which is a React component library that allows copying text to the clipboard very easily. Since it's a JavaScript library, we can use the same import approach we used in the previous iteration. The only difference is that we will make a named import and not a default import.
1/* src/App.re */
2
3[%bs.raw {|require('./App.css')|}];
4
5let countWordsInString = text => {
6 let spacesRegex = Js.Re.fromString("\s+");
7
8 switch (text) {
9 | "" => 0
10 | noneEmptyText =>
11 noneEmptyText
12 |> Js.String.trim
13 |> Js.String.splitByRe(spacesRegex)
14 |> Js.Array.length
15 };
16};
17
18module Times = {
19 [@bs.module "./icons/times.svg"] [@react.component]
20 external make: (~height: string) => React.element = "default";
21};
22
23module Copy = {
24 [@bs.module "./icons/copy.svg"] [@react.component]
25 external make: (~height: string) => React.element = "default";
26};
27
28module CopyClipboard = {
29 [@bs.module "react-copy-to-clipboard"] [@react.component]
30 external make: (~text: string, ~children: React.element) => React.element =
31 "CopyToClipboard";
32};
33
34[@react.component]
35let make = () => {
36 let (text, setText) = React.useState(() => "");
37
38 let handleTextChange = e => ReactEvent.Form.target(e)##value |> setText;
39
40 let handleClearClick = _ => setText(_ => "");
41
42 let wordsCountText =
43 (text |> countWordsInString |> string_of_int) ++ " words";
44
45 <div className="App">
46 <div className="header">
47 <h3> {"Words Counter" |> ReasonReact.string} </h3>
48 <span> {ReasonReact.string(wordsCountText)} </span>
49 </div>
50 <textarea
51 placeholder="Express yourself..."
52 value=text
53 onChange=handleTextChange
54 />
55 <div className="footer">
56 <Button
57 title="Clear text"
58 onClick=handleClearClick
59 disabled={String.length(text) === 0}>
60 <Times height="20px" />
61 </Button>
62 <CopyClipboard text>
63 <Button
64 title="Copy text"
65 disabled={String.length(text) === 0}
66 category=Button.PRIMARY>
67 <Copy height="20px" />
68 </Button>
69 </CopyClipboard>
70 </div>
71 </div>;
72};
I like
Importing a JavaScript React component library is also very simple and insures type safety.
Keep reading the next part of this series here.